I'm replying to NightWolve's question here to avoid polluting the original thread ...
Custom VWF font (with added drop-shadow) ...
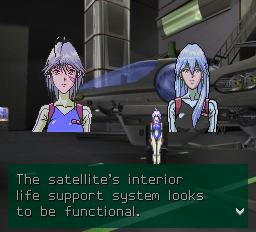
Impressive work to be sure! How did you pull that off ? Like, say, how much PC-FX assembly code did you write/mod to accomplish the task ?
I'd love to be able to say wonderful things about how smart I am in hacking a VWF into Team Innocent ... but I'm afraid that I can't.
The truth is, that what you're seeing in that screenshot isn't a "true" VWF ... it's a "BWF", a bi-width font.
The characters that are displayed are either 4x16, or 8x16.
The actual font itself is designed in such a way as to minimize the clues that would give away that fact that it isn't a "true" VWF. :-"
Now, the reason for doing this instead of a real VWF, is that Team Innocent writes all of it's strings to a "virtual" screen in 4x8 pixel chunks, and then uploads any "changes" to VRAM in a totally separate "task" that runs every-now-and-again.
I couldn't figure out how to hack a VWF into such a bizarre scheme, but it did eventually occur to me that although the code normally just prints 8-wide or 12-wide fonts (2 or 3 4-pixel chunks), there was no reason that it couldn't print single chunk wide characters (i.e. just 4-pixels).
So, the entire "VWF" hack was just telling it that certain characters should be printed with a width of 1 4-pixel chunk instead of 2 or 3 4-pixel chunks.
Then it was just a case of hacking in a custom set of font data that took advantage of the new capability.
So, the new "VWF" code is just ...
_get_glyph_width:
movea 0x3fff, r0 ,r1 // glyph $8000-$ffff (12x12)
cmp r1, r27
mov 3, r28 // width=3
bgt 1$
movea 0x1fff, r0 ,r1 // glyph $0000-$00ff (8x8)
cmp r1, r27
mov 2, r28 // width=2
ble 1$
addi -' ', r27, r1 // glyph $2000-$20ff (6x12)
andi 0x7f, r1, r1
movea lo(_step8x14v), r1, r1 // read the width (in 4-pixel chunks)
movhi hi(_step8x14v), r1, r1 // from a table.
ld.b 0[r1], r28
1$: jr _got_glyph_widthIf that looks too strange, this might help ...
V810 CPU Architecture Manual
http://www.goliathindustries.com/vb/download/cpu/U10082EJ1V0UM00.pdfNow, as to "how" I actually got to the point of knowing how the Team Innocent code was working in that strange way, and exactly where to hack in that "solution".
I'm afraid that that's just the usual boring hacking job of identifying interesting-looking memory accesses, and then tracing and hand-disassembling a few thousand instructions back into their equivalent 'C' code to understand what they're doing.

What seems a little sad, is that while Zeroigar has "proper" VWF font printing, with kerning, too ... the truth is that most people won't notice the difference.
And that was a significantly larger and much more complex piece of code to write!
I think that the big thing for anyone reading this to take away, and a good lesson that I'd totally forgotten myself, is that you sometimes don't need the "perfect" solution in order to get something that actually looks good on the screen.
